
Quest System
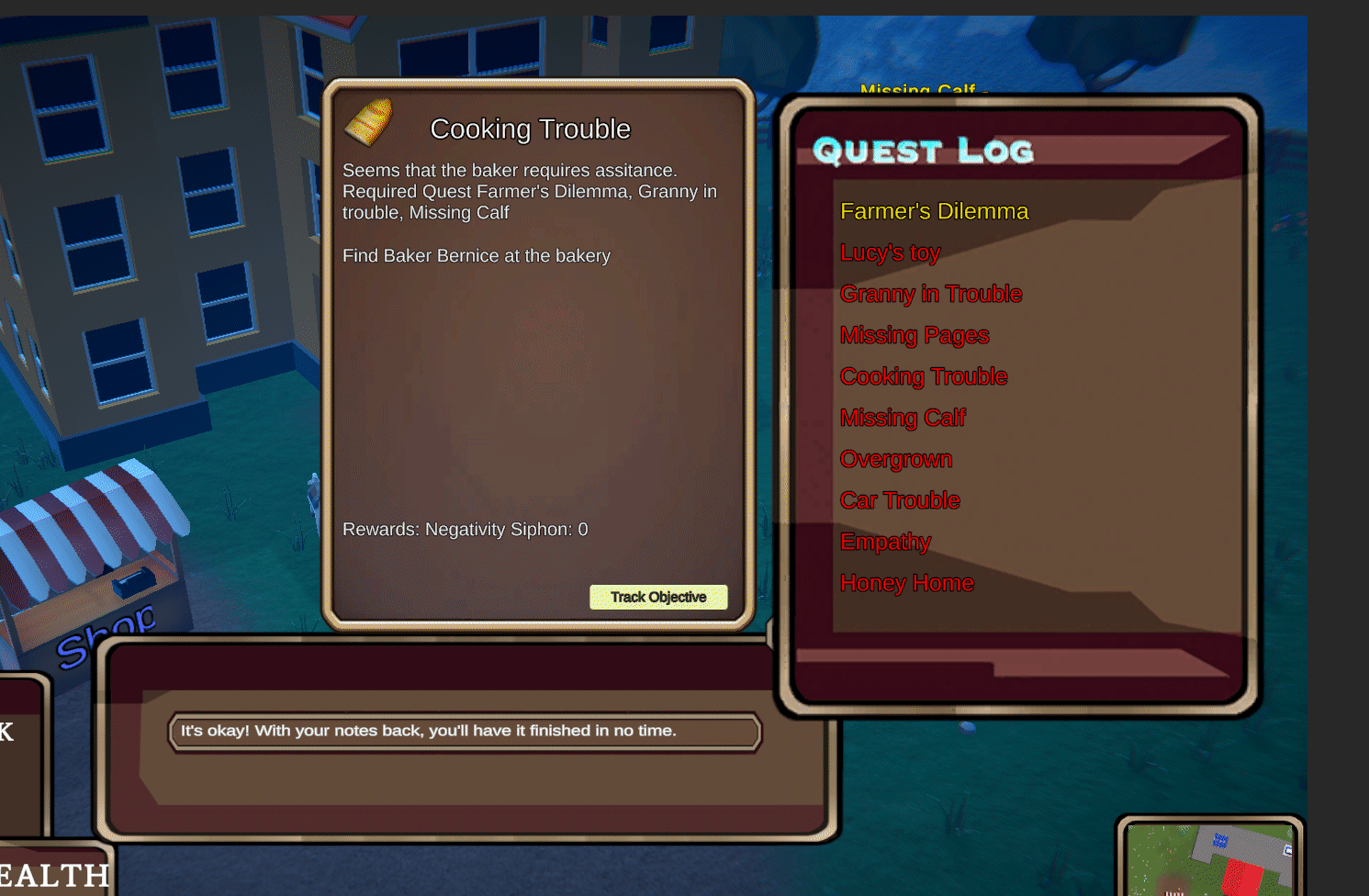
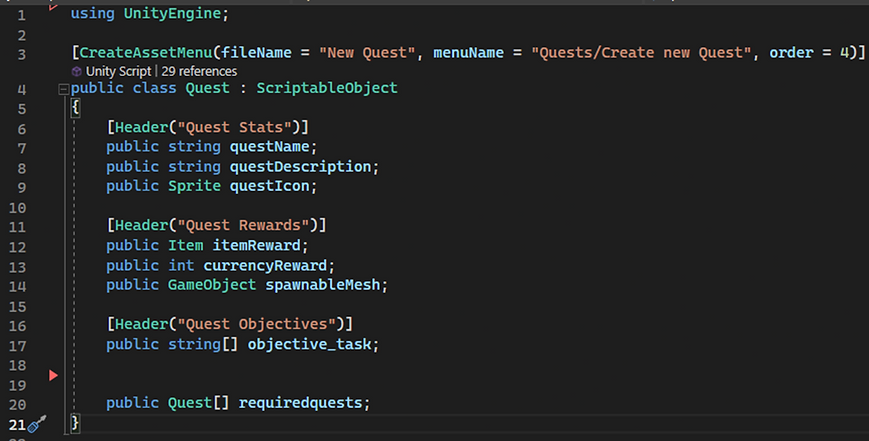
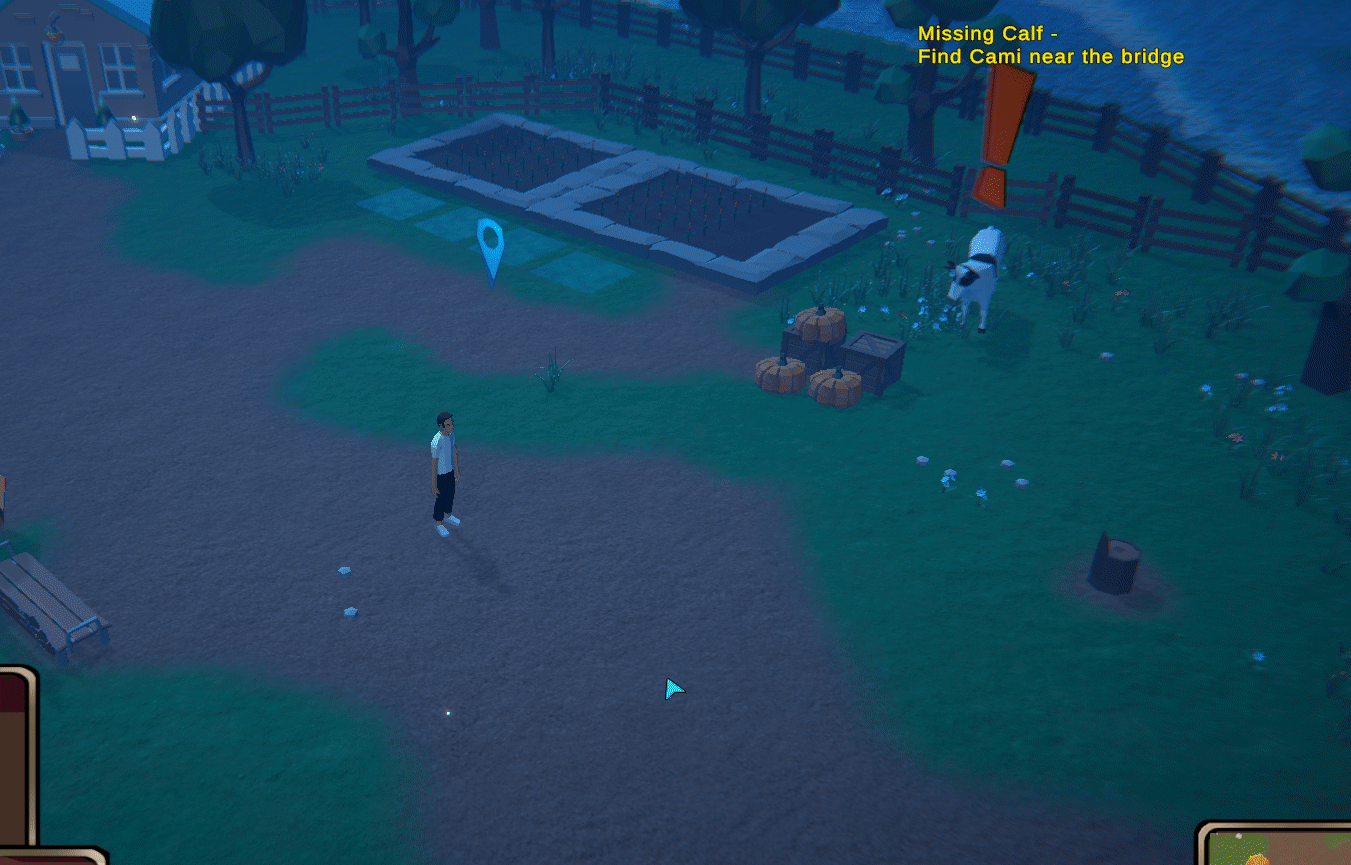
Overview
On this page, I will explain how I developed a quest system in Unity engine for the game Empathy. This system allows players to view available quests and objectives to advance in the game, and it is a frequently used feature in RPG games.
Intent
This page intends to provide information on how I implemented the quest system so developers can understand the progress in creating one.
Creating a quest and its properties
To create a quest, I created a scriptable object to store the main properties of the specific quest. This includes the name of the quest, description, the quest icon, the rewards, the objective steps, and the required quest.
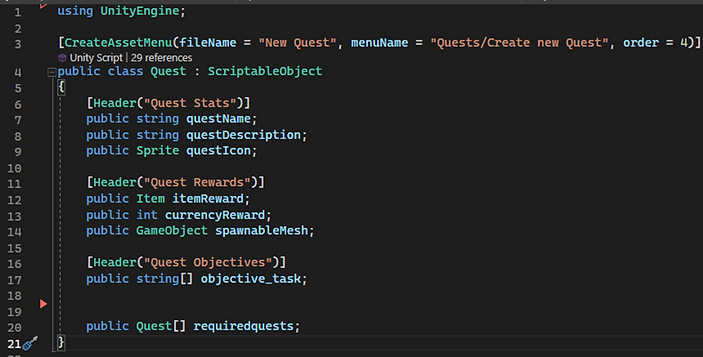
Variable Spawnable mesh
The spawn-able mesh variable keeps track of the prefab used to store the quest object which is the object that spawns with the quest journal to populate the quest information.
Objective tasks
Objective tasks indicate how often the quest should update on the quest page after completing a step. For instance, if the player needs to obtain an item for an NPC, the first step is to talk to the NPC and get the necessary information. Once the player completes this step, the quest page will display new information for the next step. This feature also enables players to save their progress at specific points in the quest, allowing them to resume that objective later.
​
Creating a quest
Once the scriptable object script for the quest is created. Developers can create their own quest data by putting values for the data asset. The developers would have to make sure the scriptable objects for the created quest data asset are within a resource folder so it can be picked up if required though scripting.
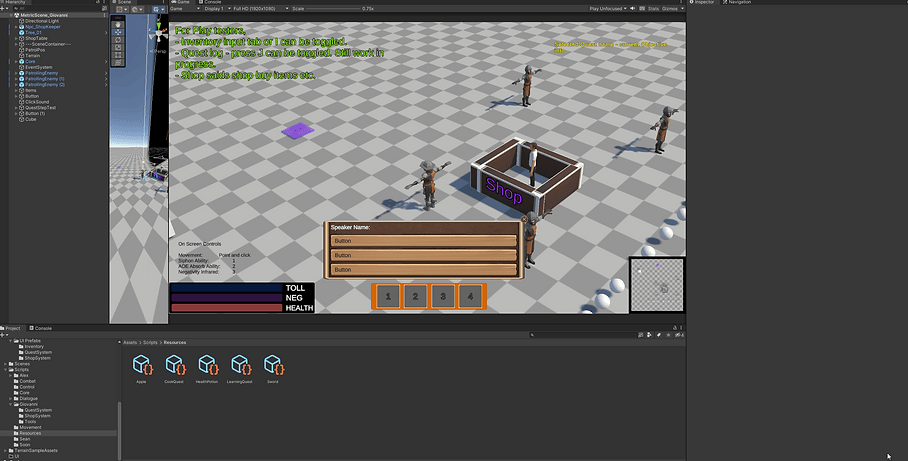
Quest Data Script
To properly save each individual quest information. I created a script called Quest Data which allows information like the color, it's in progress state, has been completed state, the current objectives, and the unique functions of that quest to be registered with other scripts.

ShowCurrentObjective( )
This function allows the quest to reference its current quest objective so it can be referenced with a button or journal which allows the current objective to be displayed within the UI for the player to see.
UpdateQuestData( )
This function updates the information for each quest on the quest log. This one is usually placed on awake or start of the quest log script.
​
Quest log or journal
This script will oversee keeping track of every quest that the game has and put it in a journal so the player can see the quest through the UI. I’ll explain the variable usage below.

Quest system base variables
Quest log - An array of Quest objects that represent the quests that can populate the quest log. This allows the quest journal to track the quest scriptable objects and their properties.
Quest Savable - An array that references the script called Quest Data that allows the quest data like the color, if it's in progress, has been completed, and the current objectives for each quest.
Questlogparent - A reference to the quest log UI object that displays all the quests.
Quest Journal UI components
Quest Click - An array of Button objects that represent the clickable buttons for each individual quest in the UI.
​
ObjectiveInScreen - A Text mesh pro text variable representing the gameplay objective displayed outside the quest menu.
​
The Quest page section of variables fills out the additional quest page that pops up when you click a specific quest in the quest journal.
​
Quest log Script Awake method
Setting up awake function. I must give the arrays a set value using the "new" keyword, so it doesn’t say the array is full. I can safely do this without cleaning up the memory in C# afterward. Then set up each individual quest to be populated with quest data and progress using a for loop.
​
​
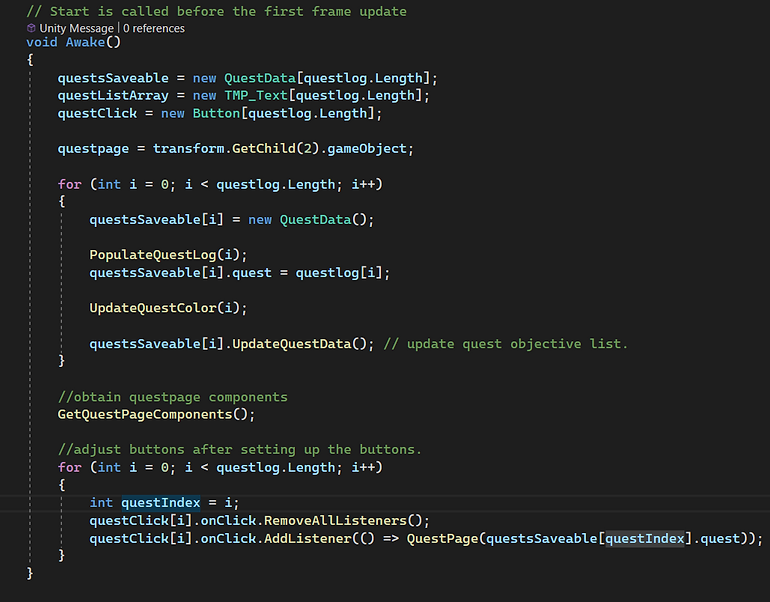
Using the for loop I created the array for each individual button for the quest to be clicked on and protected myself from the buttons having data from previously opened quest log by clearing the event listers from the buttons assigned for the quest. Then set up the buttons will the correct quest on each button index.
ShowQuestTracking()
This function is set up to display the current objective of an individual quest in the gameplay UI which is tracked by the index.
GetQuestPageComponents()
This function retrieves references to different UI components of the quest page.
​
​
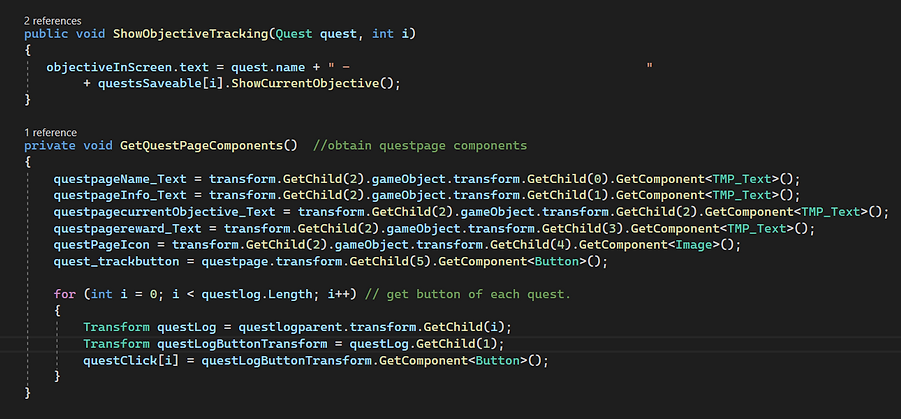
UpdateQuestColor()
The function is set up to update the color of a specific quest index in the quest log based on its progress. This is tracked using red as not started, yellow as in progress, and green as completed.
PopulateQuestLog()
Instantiates and sets up the quest log UI elements for each quest and gets the quest name to show on the quest UI display when opened. I also set up a debug color if the quest progress wasn’t set up correctly. The quest object in the quest log is organized in the UI using the grid component which automatically organizes objects placed in the parent based on set values.
​
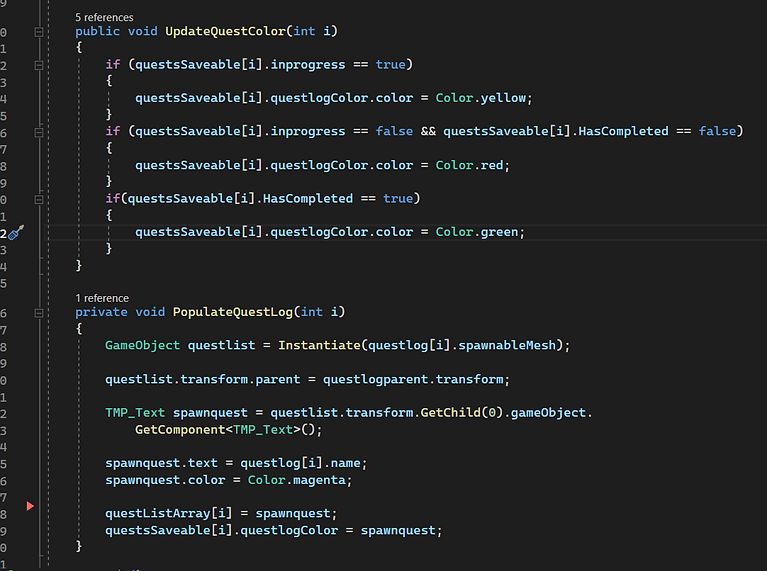
QuestPage()
The quest page is the page that opens when a quest name is clicked on the quest log. Upon clicking the quest, a quest page will open for that specific quest.
The method displays information about a specific quest index in the quest page UI that the user has selected. It also sets up the tracking button to show the current objective of the quest.
​
​
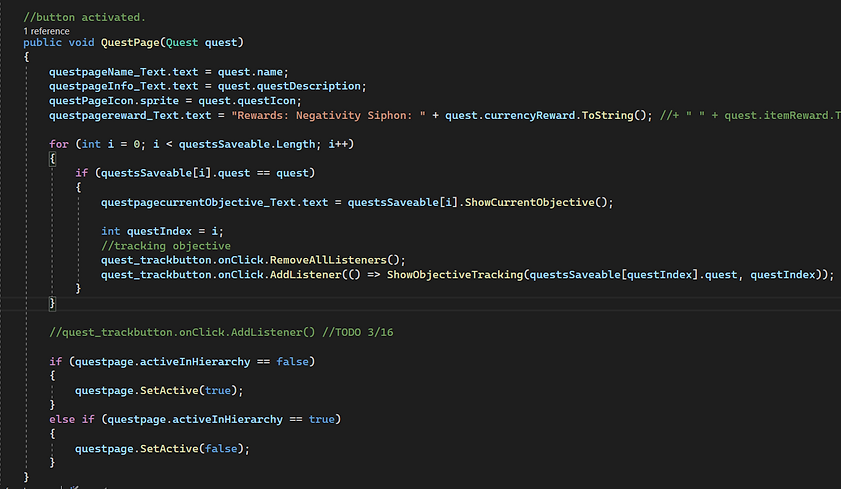
UpdateQuestProgress()
This function is usually fired when something from the quest has happened, and it needs to move to the next objective of the quest. The function obtains the correct quest index of the quest list and updates the quest objective to the next one then displays the next objective in the gameplay UI automatically for the player.
​
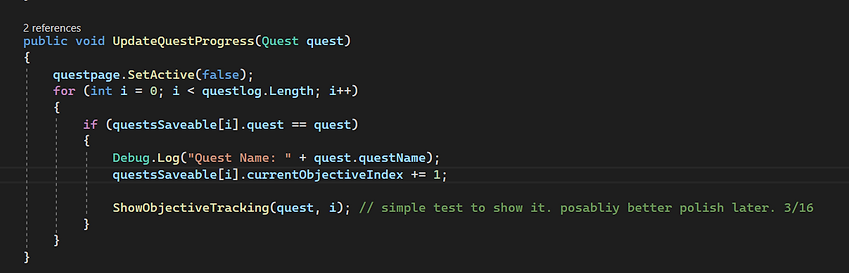
StartQuest()
This function will attempt to start the quest but before doing so will check if the required quests for starting a quest have been completed by checking the scriptable object required quest variable. If so, it will start the quest and convert the color of the quest to a yellow color showing it in progress.
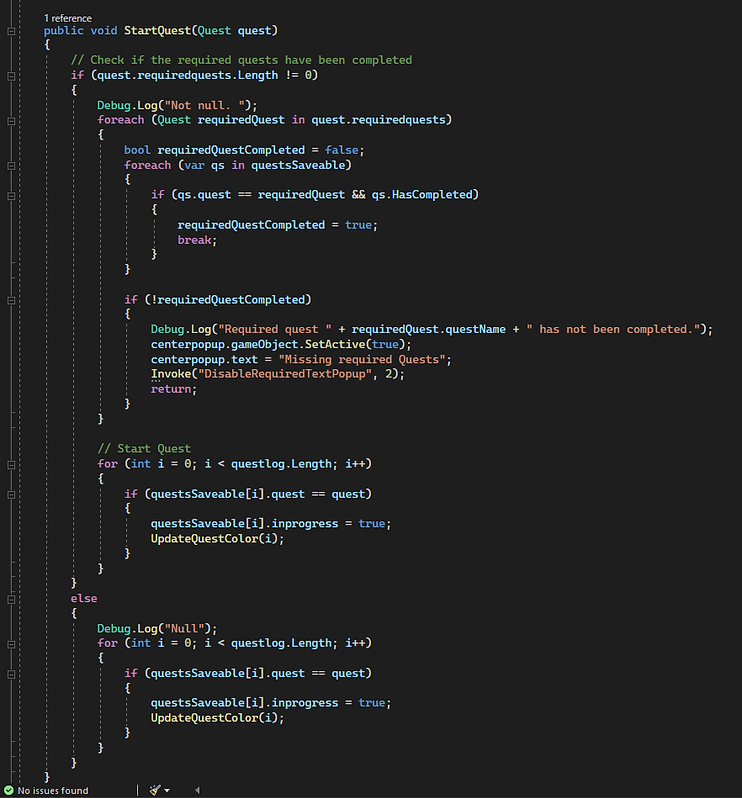
CompleteQuest()
When the function is executed, it will mark the quest index as completed and skip any objectives that were additionally added to the end. The function would also update the quest color in the quest log, play completion sound, and reward the player by reducing its negative currency based on the game instead of giving it a rewarded item right away.
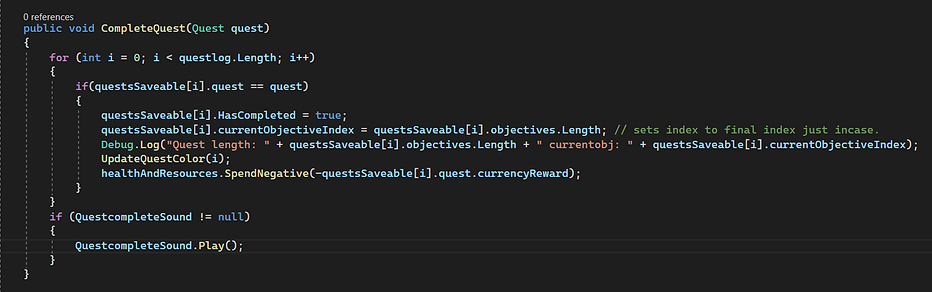